Webpack 정리노트
1. 개념
2. webpack 간단 사용법
3. 설정 파일 사용법
4. CSS 사용법 (나중에 다른 파일형식으로 응용가능)
5. ES6 사용법
6. 외부 모듈 사용법
7. 다중 output 파일 생성법
8. 파일 관리
9. asset 파일 관리
10. sourcemap 생성
11. ES7 .. 더넘어서
1. Webpack 간단 개념
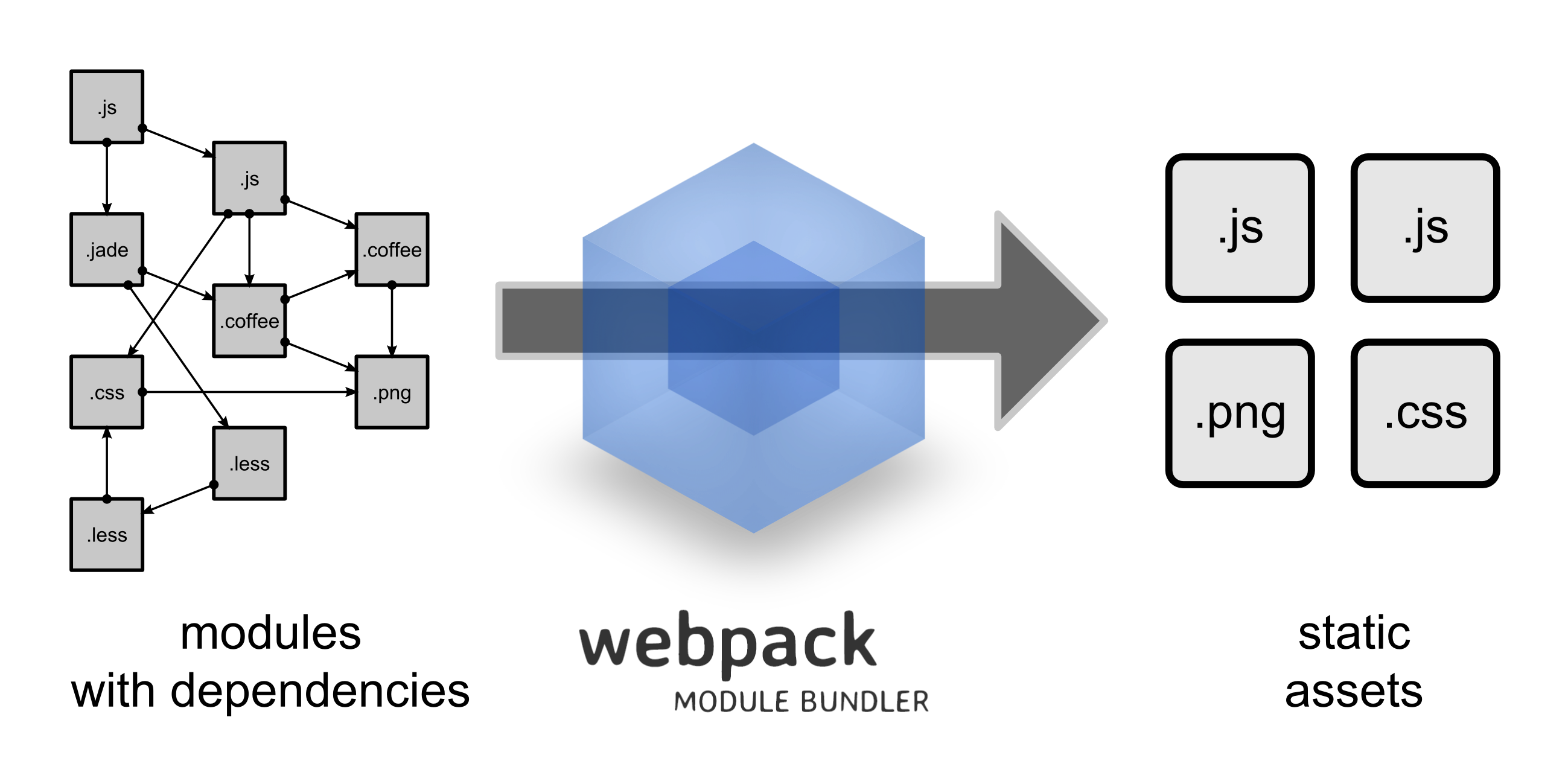
위의 그림으로 부가적으로 설명하자면 여러가지의 의존성을 가진 파일들을 모아 한가지 파일로 만들어주는 역할을 한다.
기존 browser 내 자바스크립트에서 모듈기능의 부재로 인해 많은 방법론들과 라이브러리들 탄생했는데 ( requirejs, commonjs, AMD..) 이런 문제를 해결해주는 방법의 하나로 보면 된다.
번들링하는 과정중에 transpiling 을 지원하므로 ES6 문법을 활용한 코딩도 가능하다.
자바스크립트파일뿐만 아니라 css, sass, image files 등 다른 파일들에 대한 묶음(bundle)기능도 제공한다.
- 어디에 사용해야 할까?
프론트 엔드 프레임워크와 함께 SPA 에서 프론트 엔드를 헤비하게 사용하는 곳에서 엄청난 강점을 발휘
반면 프론트 엔드 코드 베이스가 크지 않은 곳에서는 overhead로 다가올수도 있다. (설정하는데 한세월...)
이렇게 하면 사실 잘다가오지 않아서 실제적인 예를 들고자 한다.
추가 참고:
http://blog.andrewray.me/webpack-when-to-use-and-why/
2. Webpack 간단사용법
npm install webpack -g
1. 모듈 참조 파일 작성
moduleA.js
moduleB.js
entry.js
import a from "./moduleA";
import b from "./moduleB";
* npm에서 다운로드 받은 파일 (node_modules)에 있는 파일들은
import dfd from "module"; 식으로 사용 가능
2. webpack entry.js bundle.js
bundle.js 파일 생성
bundle.js안에 moduleA와 moduleB를 사용할수 있다.
[--watch]
3. 설정 파일 사용법
위의 같은 경우같이 계속해서 수동으로 파일 위치를 지정해주는 것은 비효율적이므로 webpack.config.js 설정 파일만들어 자동화가 가능하다.
기본 설정파일 구조
module.exports = {
context: path.resolve(__dirname, './src'), // 소스 디렉토리 루트 폴더 위치
entry: './entry.js',
output: {
path: __dirname,
filename: 'bundle.js'
}
};
webpack 명령어를 치면 기본적으로 webpack.config.js 파일을 찾는다. 하지만 보통 프로덕션, 테스팅, 로컬 환경에 따라 다른 파일을 쓰게 되므로
다른 설정파일을 쓰고 싶은 경우
webpack --config 설정파일경로 로 설정한다
* __dirname은 현재 폴더를 나타낸다.
4. CSS 사용법
webpack 은 javascript 모듈뿐만 아니라 css도 지원한다.
- CSS
파일안에 CSS 추가파일 참조
ES5 버전 이하
require('!style!css!./style.css');
ES6 이후
import './style.sass';
- SASS
npm install sass-loader node-sass --save-dev
require('!style!css!sass!./style.sass');
module.exports = {
entry: './entry.js',
output: {
path: __dirname,
filename: 'bundle.js'
},
module: {
loaders: [
{ test: /\.sass$/, loader: 'style!css!sass' }
]
}
};
- LESS
npm install less-loader less --save-dev
module.exports = {
module: {
loaders: [
{
test: /\.less$/,
loader: "style!css!less"
}
]
}
};
* 설정파일에 CSS 설정 추가법
5. ES6 사용법
ES6의 경우는 사용법이 더 간결하다. ES6자체 모듈 기능을 활용하자.
( ES5까지의 노가다 코딩 과정을 ES6과정을 통해 확연히 줄이자 ES6 만세! )
webpack 로더 추가
npm install --save babel-loader babel-core babel-preset-es2015
module.exports = {
entry: './entry.js',
output: {
path: __dirname,
filename: 'src/js/dist/bundle.js'
},
module: {
loaders: [
{
test: /\.js$/,
loader: 'babel',
exclude: /(node_modules|bower_components)/,
query: {
presets: ['es2015']
}
}
]
}
};
6. 외부 모듈 사용법
{
output: {
// export itself to a global var
libraryTarget: "var",
// name of the global var: "Foo"
library: "Foo"
},
externals: {
// require("jquery") is external and available
// on the global var jQuery
"jquery": "jQuery"
}
}
위와 같은 설정을 할시에 jquery 파일은 어디에 위치해 놓아야 하는가?
혹은 ES6의 경우 npm 설치시에 import 설정해주면 바로 작동된다
예를들어 jquery 사용시 파일안에
import $ from 'jquery'; 로 사용 가능
* 추가 참고:
1. https://webpack.github.io/docs/library-and-externals.html
2. http://stackoverflow.com/questions/22530254/webpack-and-external-libraries
3. http://stackoverflow.com/questions/28969861/managing-jquery-plugin-dependency-in-webpack
7. 다중 output 파일 생성법
인풋파일 (엔트리 포인트)가 여러가지일 경우 대처 (https://webpack.github.io/docs/multiple-entry-points.html)
SPA(Single Page Application) 어플케이션이 아니라 전통의 웹 어플리케이션을 확장하다보면 하나의 아웃푸 파일에 모든 파일에 집어 넣기 보다는 필요한 모듈만 바꾸어서 아우풋 파일을 내야하는 경우가 많다.
웹팩 페이지의 다중 output 파일 기본설정
{
entry: {
a: "./a",
b: "./b",
c: ["./c", "./d"]
},
output: {
path: path.join(__dirname, "dist"),
filename: "[name].entry.js"
}
}
여기서 웹팩에서
* 추가 참고:
1. https://github.com/webpack/webpack/issues/1189
2. https://webpack.github.io/docs/configuration.html
8. CSS외에 다른 로더들도 사용해보자 (스킵 가능)
필자는 C#의 창시자 엔더스 헤일즈버그를 좋아한다. 그러므로 그가 참여한 프로젝트인 Typescript 또한 주목하고 있었고 현재 프로젝트에는 적용하고 못하고 있지만 군침만흘리고 있다. webpack 에서도 typescript 및 다른 css trasnpiler 들을 알아보자. 각각의 프로젝트에 맞는 로더를 구글링해서 아래와 비슷한 형식으로 추가해주면 된다.
$ npm install ts-loader --save
module.exports = {
entry: './app.ts',
output: {
filename: 'bundle.js'
},
resolve: {
// Add `.ts` and `.tsx` as a resolvable extension.
extensions: ['', '.webpack.js', '.web.js', '.ts', '.tsx', '.js']
},
module: {
loaders: [
// all files with a `.ts` or `.tsx` extension will be handled by `ts-loader`
{ test: /\.tsx?$/, loader: 'ts-loader' }
]
}
}
typescript 는 tsconfig.json 파일을 설정파일로 사용한다.
{
"compilerOptions": {
"target": "es5",
"sourceMap": true
},
"exclude": [
"node_modules"
]
}
** exlcude 설정
exclude 를 설정하게 되면 exclude 경로안에 파일들은 제외하고 컴파일한다.
* 추가 참고:
1. http://www.jbrantly.com/typescript-and-webpack
2. https://www.typescriptlang.org/docs/handbook/react-&-webpack.html
3. https://github.com/TypeStrong/ts-loader
9. asset 파일 관리
webpack 이 이미지파일도 모듈단위로 bundling 을 할수 있다. (이쯤되면 webpack이 못하는게 뭐야?)
예제로 그림파일을 첨부하는 방법을 설명한다. 먼저 에셋 파일들을 처리할수 있는 filter-loader 를 설치하자
* 추가 참고:
10. Uglify
자바스크립트를 최소화하고 싶을때 쓰는 것.
Plugin 형태로 매우 쉽게 사용가능하다. 아래와 같이 설정 파일에 덧붙여주자
plugins: [
new webpack.optimize.UglifyJsPlugin()
]
이쯤되면 loader 와 plugin 의 차이점이 무엇인지 궁금할 것이다. (본인 스스로에서 나온 경함담..)
11. soucremap 생성법
sourcemap 은 옵션을 추가해서 간단하게 생성가능하다. Happy Javascript Debugging
{
devtool: 'source-map'
}
12. ES7
추가 참고:
http://jamesknelson.com/using-es6-in-the-browser-with-babel-6-and-webpack/
지금까지 다읽은 분들은 최종 설정파일들이 어떤식으로 궁금한지도 궁금할 것이다.
(부분 소스코드만으로는 이해하기 어려운 경우르 많이 겪었다.)
그래서 지금까지 차근 차근 읽어오면서 설정을 추가하면 아래와 같은 설정파일이 나올것이다.
** 유용한 플러그인
https://www.npmjs.com/package/webpack-fail-plugin
** 웹팩 플러그인 리스트
https://github.com/webpack/docs/wiki/list-of-plugins
전체참고:
1. https://webpack.github.io/
2. http://d2.naver.com/helloworld/0239818
3. http://hyunseob.github.io/2016/04/03/webpack-practical-guide/
4. https://medium.com/@dtothefp/why-can-t-anyone-write-a-simple-webpack-tutorial-d0b075db35ed#.qbdn1ed8y
5. https://github.com/petehunt/webpack-howto
6. https://medium.com/@rajaraodv/webpack-the-confusing-parts-58712f8fcad9#.2d2825m5u
7. https://web-design-weekly.com/2014/09/24/diving-webpack/
8. https://blog.madewithenvy.com/getting-started-with-webpack-2-ed2b86c68783#.cq6srzptu
* browserlify 유저들을 위한 webpack 으로 전환하기
http://webpack.github.io/docs/webpack-for-browserify-users.html
* gulp 와 webpack 함께 사용하기